1. Introduction to Looping in Python
Looping, a fundamental programming concept, is the repetitive execution of a sequence of instructions. In Python, looping is an essential coding skill that streamlines the processes, enhancing efficiency and productivity.
a. The Importance of Looping
Imagine having to manually execute the same set of instructions multiple times. Looping eliminates this redundancy, allowing developers to automate repetitive tasks and focus on more complex aspects of programming.
b. The Role of Looping in Python
Python employs various looping structures, such as the for loop, while loop, and nested loop. These structures enable developers to iterate over data structures, execute code based on conditions, and create intricate looping patterns.
2. The For Loop
The for loop is a looping structure that iterates over a sequence of items. It is particularly useful when dealing with data structures like lists, tuples, and strings.
a. Understanding the For Loop
The syntax of a for loop is as follows:
for variable in sequence:
# code block to execute
b. Practical Example of a For Loop
Consider the following example, which uses a for loop to print the numbers from 1 to 5:
for i in range(1, 6):
print(i)
3. The While Loop
The while loop is a looping structure that executes a block of code as long as a specified condition is true. It is ideal for situations where the number of iterations is unknown.
a. The Concept of the While Loop
The syntax of a while loop is as follows:
while condition:
# code block to execute
b. Illustrating the While Loop with an Example
Here is an example of a while loop that prints the numbers from 1 to 5:
i = 1 while i <= 5:
print(i)
i += 1
4. The Nested Loop
A nested loop is a loop within another loop. This structure allows for complex iteration patterns and is commonly used in multi-dimensional data structures.
a. The Idea Behind Nested Loops
The syntax of a nested loop involves one loop enclosed within another:
for variable1 in sequence1:
for variable2 in sequence2:
# code block to execute
b. A Demonstration of Nested Loops
Consider the following example, which uses nested loops to print a multiplication table:
for i in range(1, 4):
for j in range(1, 4):
print(f"{i} x {j} = {i * j}")
5. The Break Statement
The break statement is used to exit a loop prematurely, stopping the iteration process when a specific condition is met.
a. The Function of the Break Statement
The break statement is employed within a loop like so:
for variable in sequence:
if condition:
break
# code block to execute
b. Implementing the Break Statement in a Loop
Here is an example of a for loop that terminates when it encounters the number 3:
for i in range(1, 6):
if i == 3:
break
print(i)
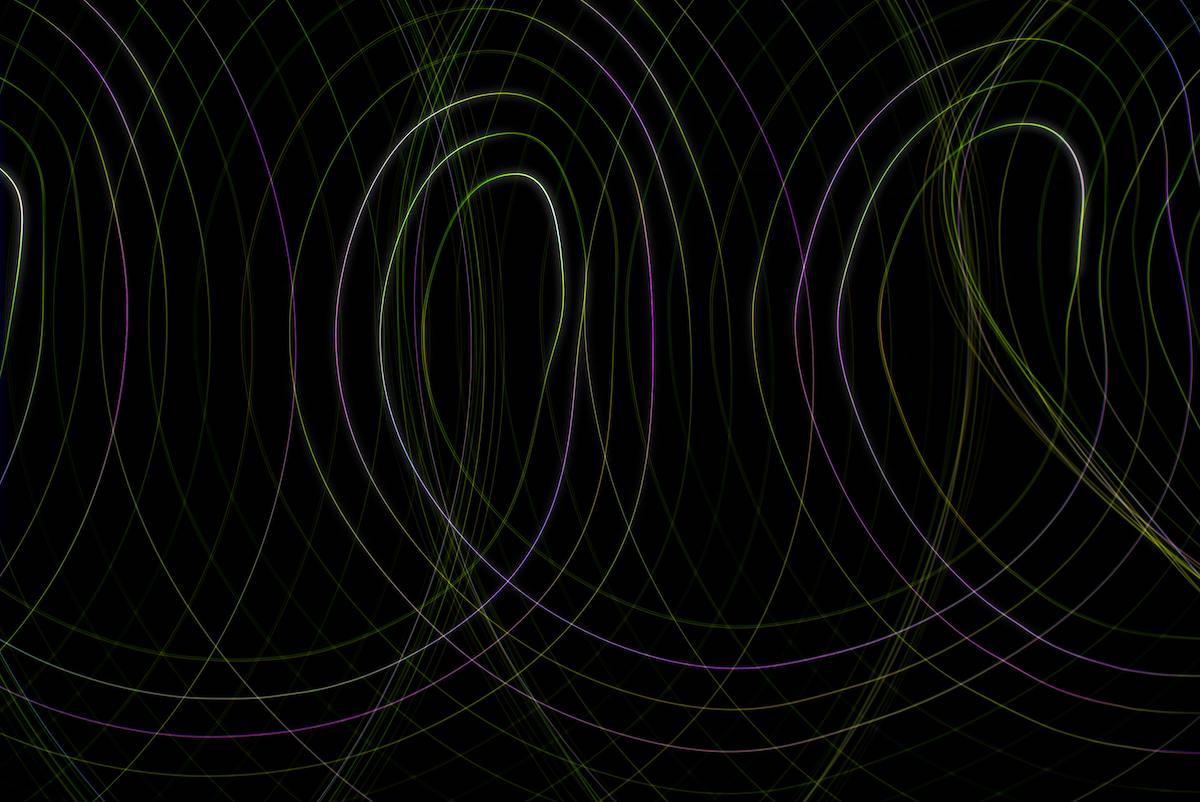
6. The Continue Statement
The continue statement is used to skip an iteration of a loop when a specific condition is met, proceeding to the next iteration without executing the remaining code in the current iteration.
a. The Purpose of the Continue Statement
The continue statement is used within a loop as follows:
for variable in sequence:
if condition:
continue
# code block to execute
b. Applying the Continue Statement in a Loop
Consider the following example, which uses a continue statement to skip printing the number 3:
for i in range(1, 6):
if i == 3:
continue
print(i)
7. The Pass Statement
The pass statement is a null operation, serving as a placeholder in situations where a statement is syntactically required, but no actual code needs to be executed.
a. The Role of the Pass Statement
The pass statement can be used within a loop like so:
for variable in sequence:
if condition:
pass
# code block to execute
b. Utilizing the Pass Statement in a Loop
Here is an example of a for loop that uses a pass statement when the number is even:
for i in range(1, 6):
if i % 2 == 0:
pass else:
print(i)
8. Conclusion: Mastering Looping in Python
Mastering looping in Python is an essential skill for any developer. By understanding and effectively utilizing various looping structures, developers can streamline their code, automate repetitive tasks, and create more efficient programs.
a. The Significance of Mastering Looping
Looping is a fundamental programming concept that underpins many aspects of software development. By honing your looping skills, you can enhance your overall coding prowess and tackle more complex programming challenges.
b. Encouragement for Further Practice
As with any skill, practice is key. Continue exploring and experimenting with Python’s looping structures to solidify your understanding and unlock your full potential as a developer. Happy coding!
Exercises
Exercise 1: Fibonacci Sequence Generator
Objective: Create a program that generates the Fibonacci sequence up to a specified number of terms using a while loop.
Instructions:
- Ask the user to input the number of terms they want to generate in the Fibonacci sequence.
- Initialize two variables,
a
andb
, to 0 and 1, respectively. These will be the first two terms of the sequence. - Use a while loop to iterate through the sequence until the desired number of terms is reached.
- In each iteration, print the current term, update the variables
a
andb
to represent the next two terms in the sequence (i.e.,a, b = b, a + b
), and increment a counter tracking the number of terms generated. - Stop the loop once the desired number of terms has been generated.
Exercise 2: Prime Number Checker
Objective: Write a program that checks if a given number is prime or not using a for loop and the break statement.
Instructions:
- Ask the user to input a number to check if it’s prime.
- Initialize a variable
is_prime
toTrue
. - Use a for loop to iterate through the numbers from 2 to the square root of the input number.
- In each iteration, check if the input number is divisible by the current iteration number. If it is, set
is_prime
toFalse
and break the loop. - After the loop, check the value of
is_prime
. If it’sTrue
, print that the input number is prime. Otherwise, print that it’s not prime.
Exercise 3: Multi-dimensional List Manipulation
Objective: Create a program that manipulates a multi-dimensional list using nested for loops.
Instructions:
- Initialize a 3×3 multi-dimensional list with integer values, such as:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
- Use nested for loops to iterate through the rows and columns of the list.
- In each iteration, multiply the current element by 2 and update the list with the new value.
- After updating all elements, use nested for loops again to print the modified multi-dimensional list in a formatted manner.
These exercises will help you practice and master various looping concepts in Python, including while loops, for loops, break statements, and nested loops. By working through these problems, you’ll gain a deeper understanding of how to effectively utilize loops in your programming projects.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good. https://accounts.binance.com/register?ref=P9L9FQKY
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Keep up the fantastic work! Kalorifer Sobası odun, kömür, pelet gibi yakıtlarla çalışan ve ısıtma işlevi gören bir soba türüdür. Kalorifer Sobası içindeki yakıtın yanmasıyla oluşan ısıyı doğrudan çevresine yayar ve aynı zamanda suyun ısınmasını sağlar.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
BWER empowers businesses in Iraq with cutting-edge weighbridge systems, ensuring accurate load management, enhanced safety, and compliance with industry standards.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article. https://www.binance.com/en-IN/register?ref=UM6SMJM3
Reading your article helped me a lot and I agree with you. But I still have some doubts, can you clarify for me? I’ll keep an eye out for your answers.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Can you be more specific about the content of your enticle? After reading it, I still have some doubts. Hope you can help me.
Your article helped me a lot, is there any more related content? Thanks!
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Your article helped me a lot, is there any more related content? Thanks!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
When I originally commented I clicked the -Notify me when new comments are added- checkbox and now each time a comment is added I get four emails with the same comment. Is there any way you can remove me from that service? Thanks!
Your article helped me a lot, is there any more related content? Thanks!
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
I discovered your weblog site on google and verify a number of of your early posts. Continue to keep up the superb operate. I simply extra up your RSS feed to my MSN Information Reader. Seeking ahead to reading more from you later on!…
I like what you guys are up also. Such smart work and reporting! Carry on the excellent works guys I’ve incorporated you guys to my blogroll. I think it’ll improve the value of my site :).
Howdy! Do you use Twitter? I’d like to follow you if that would be ok. I’m absolutely enjoying your blog and look forward to new posts.
It is perfect time to make some plans for the future and it’s time to be happy. I have read this post and if I could I want to suggest you few interesting things or advice. Maybe you can write next articles referring to this article. I wish to read more things about it!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Your article helped me a lot, is there any more related content? Thanks!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me. https://accounts.binance.com/da-DK/register-person?ref=V2H9AFPY
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Your article helped me a lot, is there any more related content? Thanks!
Your article helped me a lot, is there any more related content? Thanks!
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Your article helped me a lot, is there any more related content? Thanks!
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
магазин аккаунтов купить аккаунт
аккаунты с балансом продать аккаунт
площадка для продажи аккаунтов маркетплейс аккаунтов соцсетей
услуги по продаже аккаунтов маркетплейс аккаунтов
покупка аккаунтов маркетплейс аккаунтов
услуги по продаже аккаунтов аккаунт для рекламы
услуги по продаже аккаунтов безопасная сделка аккаунтов
гарантия при продаже аккаунтов услуги по продаже аккаунтов
профиль с подписчиками безопасная сделка аккаунтов
магазин аккаунтов магазин аккаунтов
Your article helped me a lot, is there any more related content? Thanks!
гарантия при продаже аккаунтов магазин аккаунтов
магазин аккаунтов биржа аккаунтов
купить аккаунт магазин аккаунтов
гарантия при продаже аккаунтов купить аккаунт
магазин аккаунтов социальных сетей https://pokupka-akkauntov-online.ru
Buy Account Sell Account
Secure Account Sales Buy Pre-made Account
Find Accounts for Sale Website for Buying Accounts
Marketplace for Ready-Made Accounts Sell Pre-made Account
Secure Account Sales Buy accounts
Secure Account Sales Account Selling Platform
Accounts market Buy Pre-made Account
Find Accounts for Sale Buy Account
Account Selling Platform Account Market
Buy Account Accounts market
sell pre-made account account sale
verified accounts for sale account trading platform
sell pre-made account account catalog
website for selling accounts account catalog
sell accounts https://accountsmarketbest.com/
account trading service profitable account sales
purchase ready-made accounts accounts market
verified accounts for sale verified accounts for sale
account selling platform account market
account selling service ready-made accounts for sale
account market account acquisition
account buying service online account store
purchase ready-made accounts verified accounts for sale
database of accounts for sale buy pre-made account
purchase ready-made accounts account buying service
secure account sales accounts marketplace
account buying service account trading
secure account sales buy and sell accounts
buy and sell accounts website for buying accounts
website for selling accounts sell accounts
buy pre-made account account buying platform
account trading service sell accounts
account purchase purchase ready-made accounts
secure account purchasing platform find accounts for sale
accounts market sell pre-made account
buy and sell accounts purchase ready-made accounts
account exchange account purchase
account catalog account purchase
sell pre-made account accounts marketplace
account market account marketplace
account store https://buy-best-accounts.org
find accounts for sale accounts marketplace
account buying service https://accounts-marketplace.live
sell pre-made account https://social-accounts-marketplace.xyz
buy pre-made account buy-accounts.space
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
database of accounts for sale https://buy-accounts-shop.pro/
accounts for sale https://buy-accounts.live
account buying service buy accounts
account trading platform https://accounts-marketplace.online
find accounts for sale https://accounts-marketplace-best.pro
продать аккаунт купить аккаунт
продажа аккаунтов rynok-akkauntov.top
продать аккаунт kupit-akkaunt.xyz
This article is a refreshing change! The author’s unique perspective and perceptive analysis have made this a truly engrossing read. I’m thankful for the effort he has put into creating such an informative and thought-provoking piece. Thank you, author, for providing your knowledge and igniting meaningful discussions through your exceptional writing!
маркетплейс аккаунтов соцсетей https://akkaunt-magazin.online/
площадка для продажи аккаунтов https://akkaunty-market.live/
покупка аккаунтов купить аккаунт
покупка аккаунтов https://akkaunty-optom.live
маркетплейс аккаунтов соцсетей https://online-akkaunty-magazin.xyz
купить аккаунт https://akkaunty-dlya-prodazhi.pro/
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
купить аккаунт kupit-akkaunt.online
buy facebook account buy-adsaccounts.work
buy aged facebook ads accounts buy facebook accounts cheap
buy facebook account https://buy-ad-account.top/
buy aged facebook ads accounts https://buy-ads-account.click
buy facebook account https://ad-account-buy.top
buy facebook accounts for ads https://buy-ads-account.work
buy a facebook ad account https://ad-account-for-sale.top
buy facebook old accounts buy fb account
facebook ad account for sale buy facebook ad accounts
buy account google ads https://buy-ads-account.top
buy google ads https://buy-ads-accounts.click
buy facebook account https://buy-accounts.click
buy aged google ads account https://ads-account-for-sale.top
buy google adwords accounts https://ads-account-buy.work
buy google adwords account buy google agency account
buy google ads invoice account google ads accounts for sale
buy google ads threshold account https://buy-ads-agency-account.top
buy verified google ads accounts https://sell-ads-account.click/
old google ads account for sale adwords account for sale
buy facebook bm account buy-business-manager.org
google ads reseller https://buy-verified-ads-account.work
verified bm for sale https://buy-bm-account.org/
facebook bm for sale buy verified facebook business manager
buy verified business manager buy-verified-business-manager-account.org
buy facebook ads accounts and business managers https://buy-verified-business-manager.org/
verified facebook business manager for sale https://business-manager-for-sale.org/
facebook bm account buy buy facebook verified business account
buy business manager https://buy-bm.org/
buy facebook business manager account https://verified-business-manager-for-sale.org/
buy business manager facebook buy-business-manager-accounts.org
tiktok ads account for sale https://buy-tiktok-ads-account.org
tiktok agency account for sale https://tiktok-ads-account-buy.org
buy tiktok ads https://tiktok-ads-account-for-sale.org
buy tiktok ads account https://tiktok-agency-account-for-sale.org
tiktok ads account buy https://buy-tiktok-ad-account.org
tiktok ads account buy https://tiktok-ads-agency-account.org
I’m really loving the theme/design of your website. Do you ever run into any browser compatibility issues? A couple of my blog audience have complained about my website not operating correctly in Explorer but looks great in Chrome. Do you have any ideas to help fix this issue?
What an informative and meticulously-researched article! The author’s attention to detail and capability to present intricate ideas in a comprehensible manner is truly praiseworthy. I’m totally impressed by the scope of knowledge showcased in this piece. Thank you, author, for sharing your knowledge with us. This article has been a game-changer!
buy tiktok ads account https://buy-tiktok-business-account.org
buy tiktok ads accounts https://buy-tiktok-ads.org
whoah this blog is fantastic i love reading your posts. Keep up the great work! You know, a lot of people are searching around for this information, you could aid them greatly.
I have read some good stuff here. Definitely worth bookmarking for revisiting. I wonder how much attempt you set to make the sort of fantastic informative site.
I truly appreciate this post. I have been looking all over for this! Thank goodness I found it on Bing. You’ve made my day! Thank you again
hello!,I like your writing very much! share we communicate more about your article on AOL? I require an expert on this area to solve my problem. Maybe that’s you! Looking forward to see you.
Thank you for the sensible critique. Me & my neighbor were just preparing to do a little research on this. We got a grab a book from our area library but I think I learned more from this post. I’m very glad to see such wonderful information being shared freely out there.
Oh my goodness! an amazing article dude. Thanks Nonetheless I’m experiencing difficulty with ur rss . Don?t know why Unable to subscribe to it. Is there anyone getting identical rss downside? Anybody who knows kindly respond. Thnkx
Yet another thing I would like to express is that as opposed to trying to accommodate all your online degree programs on days of the week that you finish off work (since the majority of people are worn out when they return home), try to have most of your lessons on the week-ends and only one or two courses on weekdays, even if it means taking some time off your end of the week. This is beneficial because on the saturdays and sundays, you will be much more rested along with concentrated for school work. Thanks a bunch for the different guidelines I have learned from your web site.
Your house is valueble for me. Thanks!?
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article. https://www.binance.info/sv/join?ref=PORL8W0Z
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
температура воды в хургаде в апреле
Things i have always told men and women is that when you are evaluating a good online electronics retail outlet, there are a few factors that you have to take into account. First and foremost, you should make sure to find a reputable and reliable store that has got great testimonials and scores from other customers and business sector analysts. This will make certain you are handling a well-known store to provide good services and support to its patrons. Thank you for sharing your ideas on this blog.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Hi my friend! I wish to say that this post is amazing, nice written and include almost all vital infos. I would like to see more posts like this.
I am extremely impressed with your writing skills as well as with the layout on your blog. Is this a paid theme or did you modify it yourself? Either way keep up the excellent quality writing, it?s rare to see a nice blog like this one nowadays..
красное море температура воды
I was suggested this web site by way of my cousin. I am not sure whether or not this submit is written via him as no one else recognise such designated approximately my problem. You’re incredible! Thank you!
My brother recommended I might like this website. He used to be entirely right. This put up truly made my day. You cann’t imagine just how so much time I had spent for this information! Thanks!
легальная вебкам работа в Польше Стань вебкам моделью в польской студии, работающей в Варшаве! Открыты вакансии для девушек в Польше, особенно для тех, кто говорит по-русски. Ищешь способ заработать онлайн в Польше? Предлагаем подработку для девушек в Варшаве с возможностью работы в интернете, даже с проживанием. Рассматриваешь удаленную работу в Польше? Узнай, как стать вебкам моделью и сколько можно заработать. Работа для украинок в Варшаве и высокооплачиваемые возможности для девушек в Польше ждут тебя. Мы предлагаем легальную вебкам работу в Польше, онлайн работа без необходимости знания польского языка. Приглашаем девушек без опыта в Варшаве в нашу вебкам студию с обучением. Возможность заработка в интернете без вложений. Работа моделью онлайн в Польше — это шанс для тебя! Ищешь “praca dla dziewczyn online”, “praca webcam Polska”, “praca modelka online” или “zarabianie przez internet dla kobiet”? Наше “agencja webcam Warszawa” и “webcam studio Polska” предлагают “praca dla mlodych kobiet Warszawa” и “legalna praca online Polska”. Смотри “oferty pracy dla Ukrainek w Polsce” и “praca z domu dla dziewczyn”.
легальна онлайн робота для дівчат Стань вебкам моделью в польской студии, работающей в Варшаве! Открыты вакансии для девушек в Польше, особенно для тех, кто говорит по-русски. Ищешь способ заработать онлайн в Польше? Предлагаем подработку для девушек в Варшаве с возможностью работы в интернете, даже с проживанием. Рассматриваешь удаленную работу в Польше? Узнай, как стать вебкам моделью и сколько можно заработать. Работа для украинок в Варшаве и высокооплачиваемые возможности для девушек в Польше ждут тебя. Мы предлагаем легальную вебкам работу в Польше, онлайн работа без необходимости знания польского языка. Приглашаем девушек без опыта в Варшаве в нашу вебкам студию с обучением. Возможность заработка в интернете без вложений. Работа моделью онлайн в Польше — это шанс для тебя! Ищешь “praca dla dziewczyn online”, “praca webcam Polska”, “praca modelka online” или “zarabianie przez internet dla kobiet”? Наше “agencja webcam Warszawa” и “webcam studio Polska” предлагают “praca dla mlodych kobiet Warszawa” и “legalna praca online Polska”. Смотри “oferty pracy dla Ukrainek w Polsce” и “praca z domu dla dziewczyn”.
Your article helped me a lot, is there any more related content? Thanks!
I have learned a number of important things as a result of your post. I will also like to state that there may be a situation where you will make application for a loan and do not need a cosigner such as a National Student Aid Loan. But when you are getting credit through a conventional loan provider then you need to be able to have a co-signer ready to assist you to. The lenders will probably base any decision on the few elements but the main one will be your credit history. There are some financial institutions that will in addition look at your work history and make up your mind based on this but in most cases it will be based on on your ranking.
Your article helped me a lot, is there any more related content? Thanks!
What?s Happening i’m new to this, I stumbled upon this I’ve found It positively helpful and it has helped me out loads. I hope to contribute & aid other users like its helped me. Good job.
температура воды в хургаде в феврале
система дренажа https://stroitelnoe-vodoponizhenie6.ru/ .
ai therapy chatbot https://ai-therapist1.com/ .
водопонижение в мо https://www.stroitelnoe-vodoponizhenie6.ru .
Hiya, I am really glad I have found this information. Today bloggers publish just about gossips and internet and this is really annoying. A good site with interesting content, this is what I need. Thanks for keeping this website, I’ll be visiting it. Do you do newsletters? Cant find it.
ai therapist ai-therapist1.com .
Magnificent web site. A lot of useful info here. I am sending it to several pals ans also sharing in delicious. And of course, thank you to your sweat!
Hi there! I know this is kinda off topic but I was wondering if you knew where I could get a captcha plugin for my comment form? I’m using the same blog platform as yours and I’m having trouble finding one? Thanks a lot!
Hello there! Do you know if they make any plugins to help with Search Engine Optimization? I’m trying to get my blog to rank for some targeted keywords but I’m not seeing very good gains. If you know of any please share. Many thanks!
Woah! I’m really digging the template/theme of this blog. It’s simple, yet effective. A lot of times it’s challenging to get that “perfect balance” between superb usability and visual appearance. I must say you’ve done a superb job with this. Also, the blog loads very fast for me on Internet explorer. Exceptional Blog!
ai therapist chat http://ai-therapist6.com/ .
mental health ai chatbot https://mental-health1.com/ .
I simply couldn’t leave your site before suggesting that I really enjoyed the standard info an individual provide on your guests? Is gonna be back incessantly in order to check out new posts
ai therapist bot ai-therapist6.com .
mental health support chat http://www.mental-health1.com/ .
Hello there, just became aware of your blog through Google, and found that it’s truly informative. I?m going to watch out for brussels. I will be grateful if you continue this in future. Numerous people will be benefited from your writing. Cheers!
I’m truly enjoying the design and layout of your site. It’s a very easy on the eyes which makes it much more pleasant for me to come here and visit more often. Did you hire out a designer to create your theme? Fantastic work!
I have learn a few just right stuff here. Certainly price bookmarking for revisiting. I surprise how a lot effort you put to create this type of fantastic informative website.
obviously like your website however you have to take a look at the spelling on quite a few of your posts. Many of them are rife with spelling issues and I to find it very troublesome to inform the truth however I will definitely come again again.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.