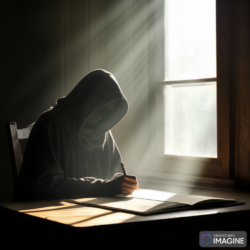
Introduction
Welcome to the exciting world of data visualization using Python. Data visualization finds applications across various domains, aiding in better comprehension and decision-making. In business, it helps identify market trends, track sales performance, and optimize strategies. It is one of key component of data science. In retail it is helping in optimizing the customer experience. In healthcare, it facilitates disease monitoring, patient care analysis, and medical research. Education benefits from visualizing student performance, learning outcomes, and curriculum effectiveness. Government agencies utilize it for public policy analysis, resource allocation, and urban planning. Moreover, data visualization plays a crucial role in scientific research, financial analysis, social media analytics, and more, making complex data accessible and actionable across diverse fields.
In this post, we’ll uncover the magic of transforming raw data into captivating charts and graphs. By the end, you’ll be equipped to unravel trends, patterns, and relationships within your data like a pro.
Let’s kick things off with a quick overview:
Unraveling Data
What is Data?
Imagine that you own a diary where you are going to write down how much money you are spending each day. So you will make multiple entries into your diary regarding your expenditure. Your entries may look like the following:
1. Monday: $5 for lunch
2. Tuesday: $10 for movie tickets
3. Wednesday: $ 15 for dinner at a restaurant
In this example each entry represents a piece of information. This collection of information is called data. Here your entire week’s spending is recorded in your notebook would be considered data. So, data is just facts or details that we can use to learn or make decisions. Just think of data as pieces of information that we can gather and/or record.
The importance of data can be gauged by its importance to help us understand patterns, trends, or relationships. By analyzing data, we can discover insights that allow us to make informed decisions. For example if we review the spending data over several weeks, we can observe different patterns such as spending more on weekends or allocating more budget to entertainment. This insight or information is very valuable and can help in effective budgeting and spending adjustments.
To summarize, data is the building blocks of information. It provide us with valuable insights that can be used to learn, understand, and make decisions in various aspects of our lives.
Data Types: The Quantitative Edge
There are many data types but the most used data type for analytics and insights is the quantitative data. In this blog we are only concerned with this type of data. Quantitative data allows for mathematical calculations, statistical analysis, and the identification of patterns and trends. Quantitative data consists of counting or numerical measurements. It provides a precise and structured format for analysis.
We can perform various statistical techniques such as regression analysis, hypothesis testing, and clustering on quantitative data. By employing these techniques, we can find relationships between variables, make predictions, and derive insights from the data.
The Power of Visualization
Data visualization isn’t just about pretty charts—it’s about clarity. With Python’s robust ecosystem of visualization libraries, we can communicate complex information in a breeze. Whether it’s spotting trends, outliers, or correlations, visualization brings data to life.
Installing Libraries: Building Blocks of Visualization
Before we dive into coding, let’s set the stage. First up, make sure you have Python installed. Then, pick your weapon of choice—be it Visual Studio Code, PyCharm, or Jupyter Notebook. Feeling adventurous? Consider creating a virtual environment to keep things tidy.
3 Examples to Kickstart Your Journey
Alright! Are you ready to flex your visualization muscles? Here are three Python scripts to get you started:
Example 1: Line Chart
The following example creates a simple line chart to visualize temperature data over time:
import matplotlib.pyplot as plt # Import library
temperatures = [20, 22, 25, 23, 21, 19] # Sample temperature data
days = [“Mon”, “Tue”, “Wed”, “Thu”, “Fri”, “Sat”]
plt.plot(days, temperatures) # Create the line chart
plt.xlabel(“Day”) # Add labels and title
plt.ylabel(“Temperature (°C)”)
plt.title(“Weekly Temperature Variation”)
plt.show() # Display the chart
Here is how it appears in Jupyter Notebook:
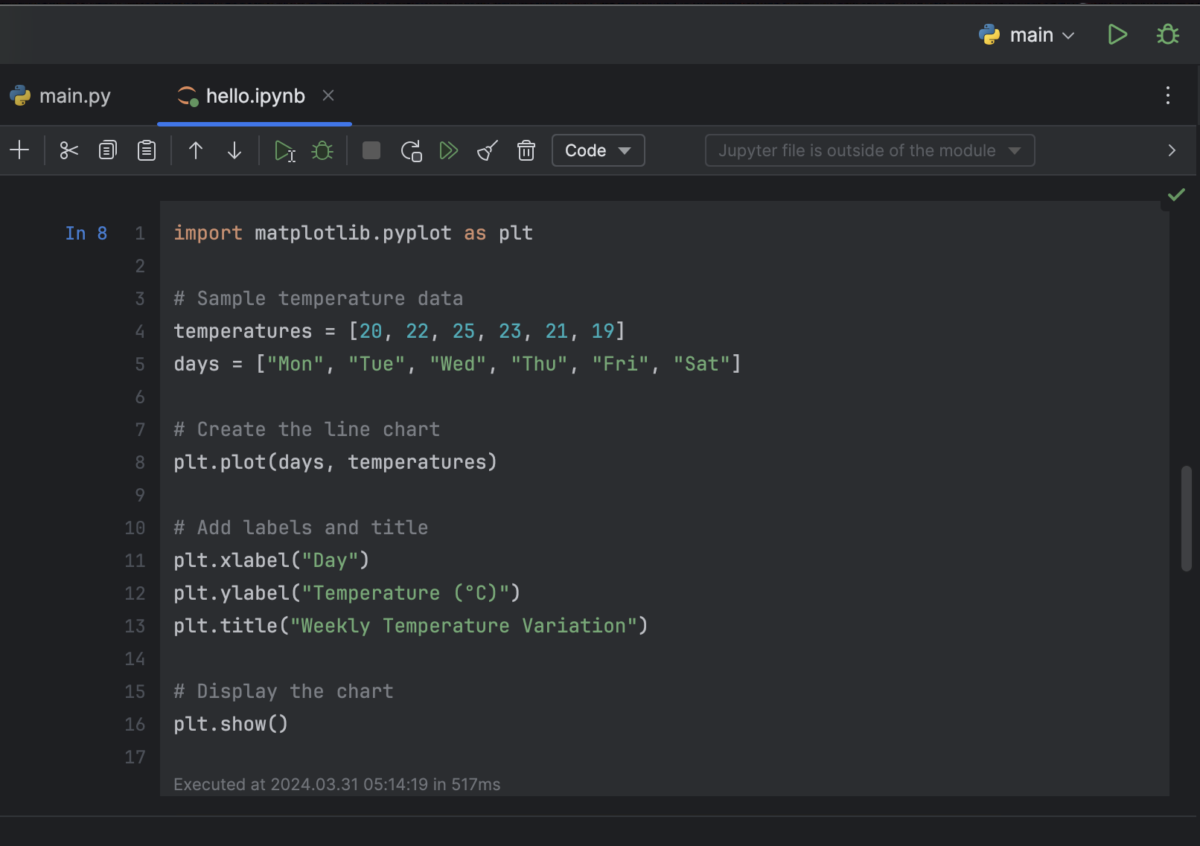
When you run the above script, you get a sleek line chart to visualize temperature variations over the week.
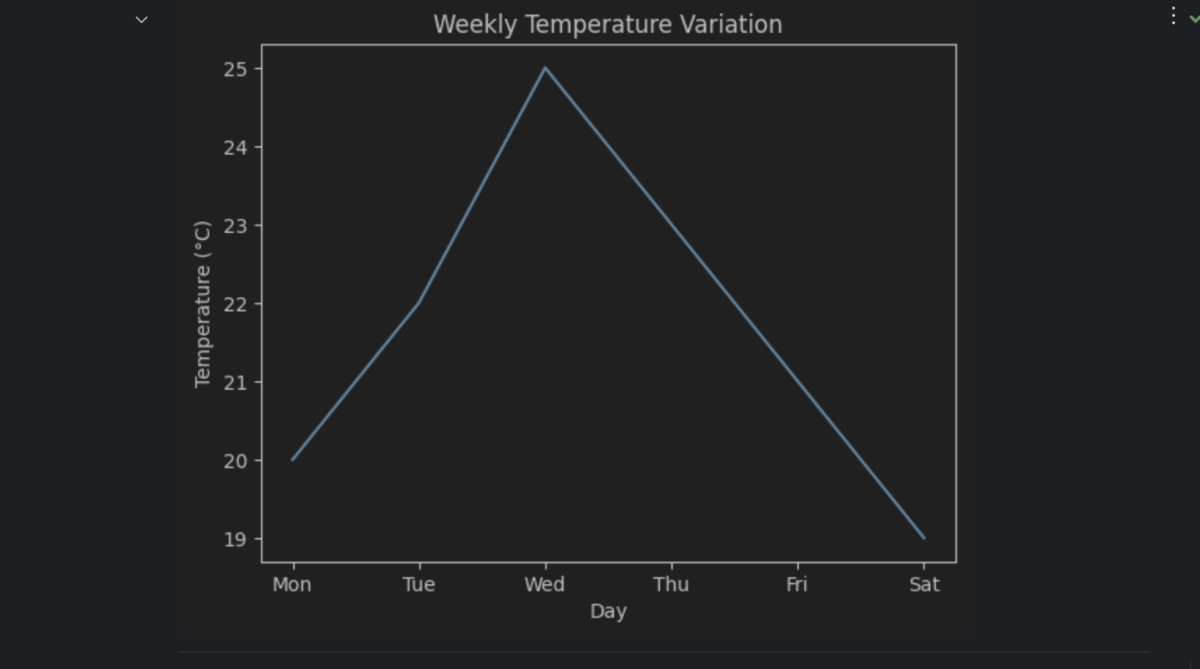
The above given script creates a line chart to visualize temperature data over different days. Here’s a detailed explanation of each line:
1. Importing the Library:
Python’s Matplotlib Library
Python/Jupyter Notebook
import matplotlib.pyplot as plt
This line imports the matplotlib.pyplot library, which is widely used for generating various types of charts and graphs in Python. We alias it as plt for easier access throughout the code.
2. Defining Sample Temperature Data:
Python/Jupyter Notebook
temperatures = [20, 22, 25, 23, 21, 19]
Here, we define a list named temperatures containing numerical values, representing the temperature readings (in Celsius, assumed) for each day.
3. Assigning Days of the Week:
Python/Jupyter Notebook
days = [“Mon”, “Tue”, “Wed”, “Thu”, “Fri”, “Sat”]
This line creates another list called days, which holds strings representing the days of the week corresponding to the temperature readings.
4. Creating the Line Chart:
Python/Jupyter Notebook
plt.plot(days, temperatures)
In this line, we use plt.plot() to create the line chart. It takes two arguments:
-
- days: The days of the week, which serve as the x-axis data points.
-
- temperatures: The temperature readings, acting as the y-axis data points.
This function connects the data points with lines, forming the line chart.
5. Adding Labels and Title:
Python/Jupyter Notebook
plt.xlabel(“Day”) plt.ylabel(“Temperature (°C)”) plt.title(“Weekly Temperature Variation”)
These lines set labels and a title for the chart:
-
- plt.xlabel(“Day”): Adds a label “Day” to the x-axis.
-
- plt.ylabel(“Temperature (°C)”): Adds a label “Temperature (°C)” to the y-axis.
-
- plt.title(“Weekly Temperature Variation”): Sets the title of the chart to “Weekly Temperature Variation”.
-
- Displaying the Chart:
Python/Jupyter Notebook
plt.show()
Finally, plt.show() is called to display the generated line chart on the screen.
Example 2: Bar Chart
Now let’s dive into customer age distribution with a snazzy bar chart powered by Seaborn:
import matplotlib.pyplot as plt
categories = [‘Category 1’, ‘Category 2’, ‘Category 3’, ‘Category 4’] # Sample data
values = [20, 35, 30, 25]
plt.bar(categories, values, color=’skyblue’) # Create the bar chart
plt.xlabel(‘Categories’) # Add labels and title
plt.ylabel(‘Values’)
plt.title(‘Bar Chart Example’)
plt.show() # Show the plot
Here is how it appears in Jupyter Notebook:
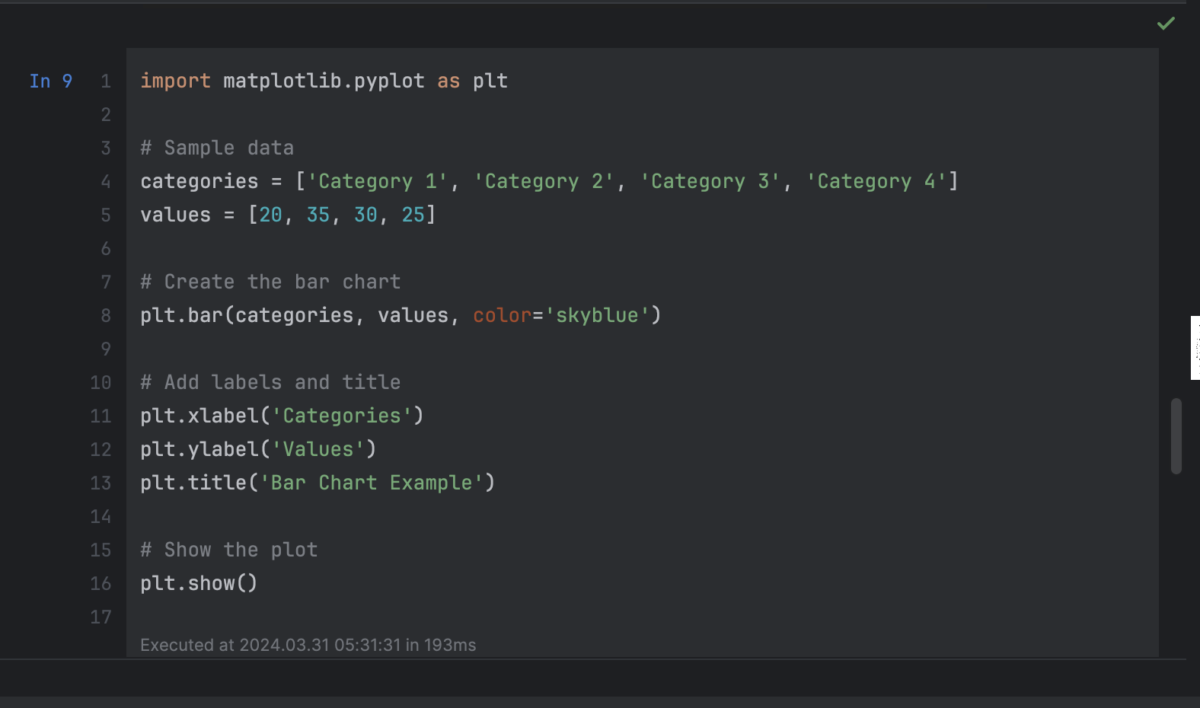
When you run the above script, you get a beautiful bar chart:
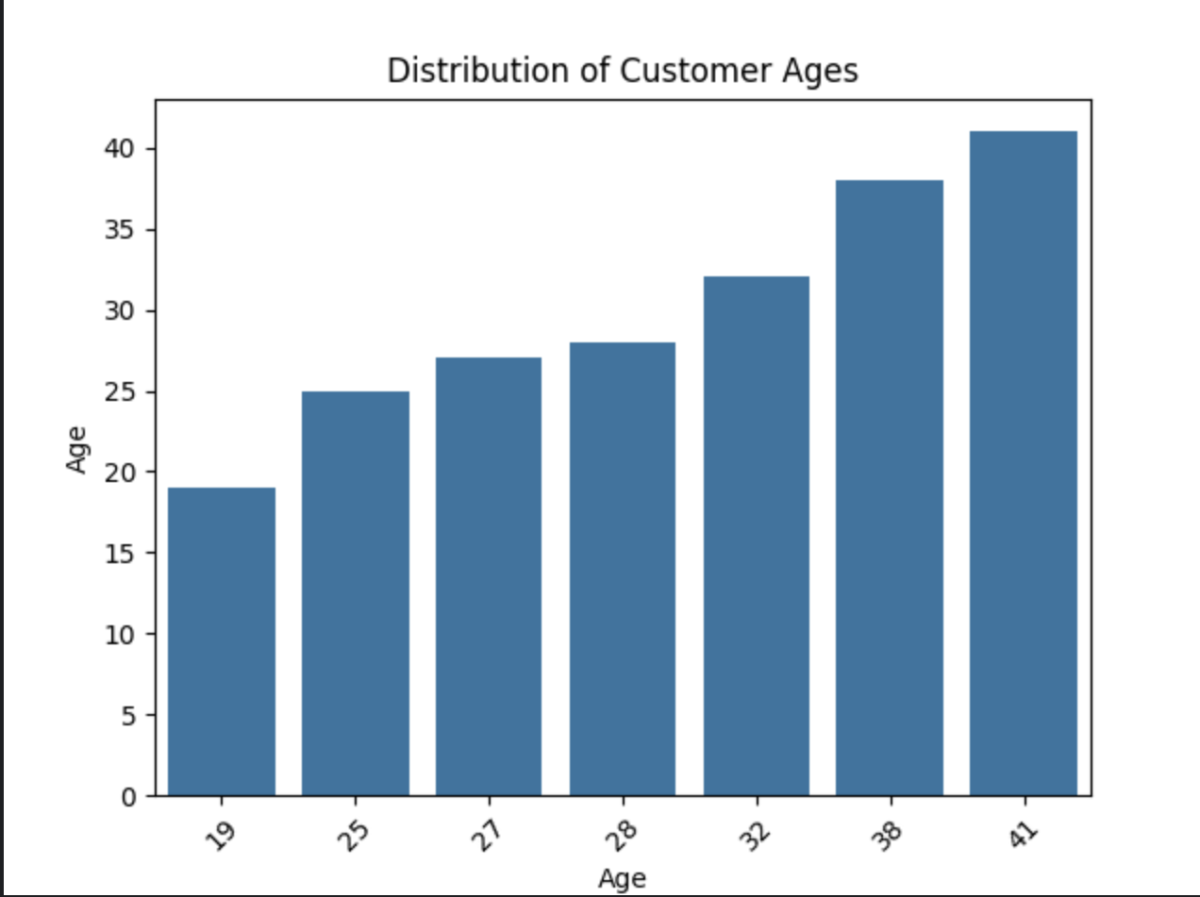
Here, we are performing data visualization in Python to represent customer age distribution using a bar chart. Here’s an explanation of the purpose of each line of our code:
1. Import Library:
Python/Jupyter Notebook
import matplotlib.pyplot as plt
This line imports the plotting functionalities from the matplotlib library and assigns it the alias plt for convenience. Matplotlib is a powerful library for creating various visualizations in Python.
2. Define Data:
Python/Jupyter Notebook
categories = [‘Category 1’, ‘Category 2’, ‘Category 3’, ‘Category 4’] # Sample data
values = [20, 35, 30, 25]
These lines define two lists:
-
- categories: This list contains labels for the bars on the x-axis (horizontal axis).
-
- values: This list contains numerical values corresponding to each category. These values represent the height of each bar in the chart.
3. Create the Bar Chart:
Python/Jupyter Notebook
plt.bar(categories, values, color=’skyblue’)
This line is the heart of creating the bar chart. Here’s what it does:
-
- plt.bar(categories, values): This method from matplotlib creates a bar chart. It takes two arguments:
-
- categories: The list of labels for the x-axis.
-
- values: The list of numerical values for the height of each bar.
-
- plt.bar(categories, values): This method from matplotlib creates a bar chart. It takes two arguments:
-
- color=’skyblue’: This sets the color of the bars to “skyblue”. You can change this value to any other color name or hex code for customization.
4. Add Labels and Title:
Python/Jupyter Notebook
plt.xlabel(‘Categories’) plt.ylabel(‘Values’) plt.title(‘Bar Chart Example’)
These lines add labels and a title to the chart for better understanding:
-
- plt.xlabel(‘Categories’): This adds a label “Categories” to the x-axis.
-
- plt.ylabel(‘Values’): This adds a label “Values” to the y-axis (vertical axis).
-
- plt.title(‘Bar Chart Example’): This sets the title of the chart to “Bar Chart Example”.
5. Display the Chart:
Python/Jupyter Notebook
plt.show()
This line tells matplotlib to display the created chart on your screen.
By running this code, you’ll see a bar chart with categories on the x-axis, values on the y-axis, a skyblue color for the bars, and the title “Bar Chart Example”.
Example 3: Histogram
This example utilizes Matplotlib to visualize the distribution of exam scores using a histogram.
import matplotlib.pyplot as plt # Import library
import numpy as np # Assuming you have exam scores as a numpy array
exam_scores = np.random.randint(60, 100, size=20) # Generate random sample exam scores
plt.hist(exam_scores) # Create the histogram
plt.xlabel(“Exam Score”) # Add labels and title
plt.ylabel(“Number of Students”)
plt.title(“Distribution of Exam Scores”)
plt.show() # Display the histogram
Here is how it appears in Jupyter Notebook:
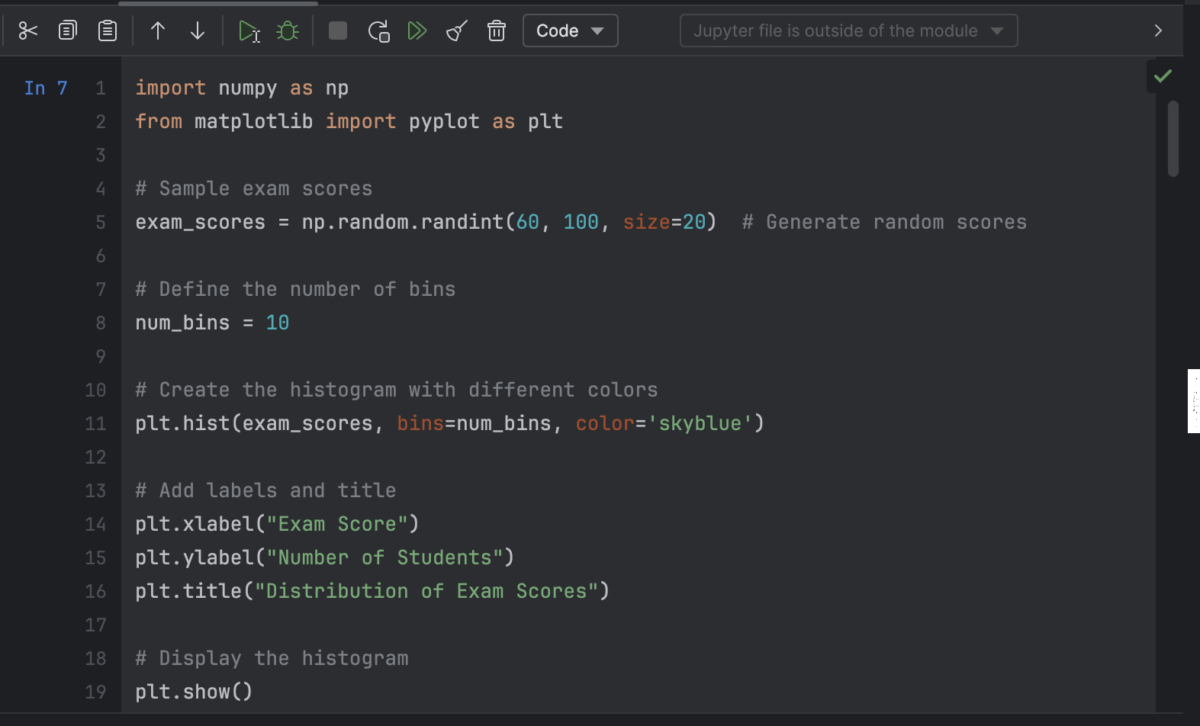
Following is how it appears when you run the code in Jupyter Notebook:
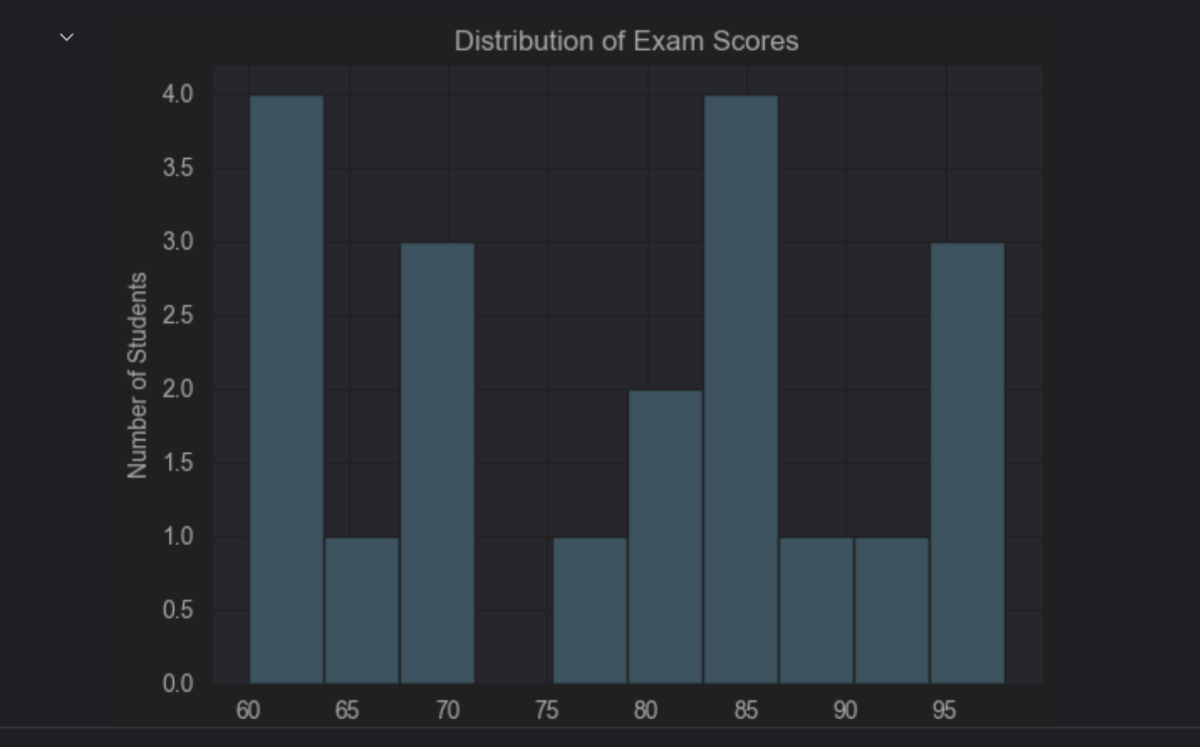
Here’s a breakdown of what the code does:
1. Importing Libraries:
-
- import matplotlib.pyplot as plt: Imports the Matplotlib library, a powerful tool for creating visual representations of data in Python. The as plt part gives it a shorter alias for convenience.
-
- import numpy as np: Imports the NumPy library, which offers efficient array manipulation and mathematical operations for numerical data.
2. Generating Sample Data:
-
- exam_scores = np.random.randint(60, 100, size=20): Creates a NumPy array called exam_scores containing 20 randomly generated integers between 60 and 100 (inclusive), simulating hypothetical exam scores.
3. Creating the Histogram:
-
- plt.hist(exam_scores): Generates a histogram based on the values in the exam_scores array. A histogram visualizes the distribution of numerical data by dividing the data range into intervals (bins) and showing the frequency of values within each bin.
4. Adding Labels and Title:
-
- plt.xlabel(“Exam Score”): Sets the label for the x-axis as “Exam Score” to clarify what the horizontal axis represents.
-
- plt.ylabel(“Number of Students”): Sets the label for the y-axis as “Number of Students” to indicate the frequency of scores.
-
- plt.title(“Distribution of Exam Scores”): Assigns a title to the plot, “Distribution of Exam Scores”, for better readability and context.
5. Displaying the Histogram:
-
- plt.show(): Renders the created histogram visually, allowing you to see the distribution of the exam scores.
Hence, we generate a histogram to visualize the distribution of hypothetical exam scores. It helps to understand the overall performance patterns of students in a more intuitive way.
Comments (3)