1. Introduction
Control flow statements play a pivotal role in dictating the execution flow of a program. In Python, they empower programmers to make decisions, iterate over data, and handle errors effectively. Understanding control flow statements is fundamental for mastering Python programming.
2. Conditional Statements
Conditional statements allow programmers to execute specific blocks of code based on the evaluation of conditions. The if
statement is a foundational component of Python’s control flow, enabling programmers to execute code selectively based on the truthiness of a condition. Additionally, the elif
and else
statements provide alternative execution paths when multiple conditions need to be evaluated.
+-----------------------+
| Conditional Statements |
+-----------------------+
|
v
Yes/No Decision
|
v
+---------+---------+
| | |
| Condition True | Condition False |
| | |
+---------+---------+
| |
v v
+-------------------+
| Execute Block |
+-------------------+
|
v
+-------------------+
| End |
+-------------------+
Conditional statements in Python allow programmers to execute specific blocks of code based on the evaluation of conditions. Let’s explore the process of utilizing conditional statements in Python.
2.1 Yes/No Decision: Assessing Condition Evaluation
At the outset, the program faces a pivotal decision – whether a condition evaluates to true or false. This initial branching point determines which path the program should take, based on the outcome of the condition evaluation.
2.2 Condition True: Executing Block of Code
If the condition evaluates to true, the program proceeds to execute the block of code associated with the true condition. This block of code contains the instructions that the program should follow when the condition is met.
2.3 Condition False: Alternative Execution Path
Conversely, if the condition evaluates to false, the program follows an alternative execution path. In this scenario, the program executes a different block of code, which contains instructions tailored to handle the situation when the condition is not met.
2.4 Execute Block: Implementation of Logic
As the program traverses through the conditional statements, it executes the appropriate block of code based on the condition evaluation. Each block of code contains instructions that dictate the actions the program should take, leading it closer to its intended objective.
2.5 End: Culmination of Conditional Execution
Upon completion of the conditional statements, the program reaches its conclusion. At this juncture, the conditional logic has served its purpose, and the program proceeds to terminate its execution, having accomplished its goals through the power of conditional evaluation.
Conditional statements in Python empower programmers to make decisions within their code, allowing for dynamic and responsive behavior based on varying conditions. By leveraging conditional statements effectively, Python programs can adapt to changing circumstances, handle different scenarios, and execute logic with precision and accuracy.
# Define a variable
age = 18
# If statement: Condition True
if age >= 18:
print("You are eligible to vote.")
# Elif statement: Alternative Execution Path
elif age < 0:
print("Age cannot be negative.")
# Else statement: Condition False
else:
print("You are not eligible to vote.")
# End: Culmination of Conditional Execution
print("Program execution ends here.")
In the above example, we first define a variable age
. The if
statement checks if the age
is greater than or equal to 18. If the condition is true, it prints “You are eligible to vote.” If the condition is false, it checks the next condition in the elif
statement. If the elif
condition is also false, it moves to the else
statement and prints “You are not eligible to vote.” Finally, the script prints “Program execution ends here.” to signify the end of the program.
3. Looping Structures
Looping structures in Python facilitate the repetition of code blocks, enabling programmers to iterate over sequences or perform tasks iteratively. The for
loop is commonly used to iterate over elements of a sequence, such as lists or tuples, while the while
loop executes a block of code repeatedly as long as a specified condition remains true.
+-----------------------+
| Looping Structures |
+-----------------------+
|
v
Yes/No Decision
|
v
+---------+---------+
| | |
| Loop | No Loop |
| Needed | |
| | |
+---------+---------+
| |
v v
+-------------------+
| Perform Loop |
+-------------------+
|
v
+-------------------+
| End |
+-------------------+
Looping structures in Python facilitate the repetition of code blocks, enabling developers to iterate over sequences or perform tasks iteratively. Let’s explore the process of utilizing looping structures in Python.
3.1 Yes/No Decision: Determining Loop Necessity
At the outset, the program faces a pivotal decision – whether a loop is needed or not. This initial branching point determines whether the program should proceed with repetitive execution based on a given condition.
3.2 Loop Needed: Initiating Iteration
If a loop is deemed necessary, the program proceeds to initiate the iterative process. Here, control flow structures such as for
loops or while
loops are employed to execute a specified block of code repeatedly, either for a predetermined number of iterations or until a certain condition is met.
3.3 Perform Loop: Executing Iterative Process
As the program traverses through the looping structure, the specified block of code is executed iteratively. Each iteration brings the program closer to its objective, as it processes data, performs calculations, or carries out other tasks as defined within the loop.
3.4 End: Culmination of Looping
Upon completion of the iterative process, the program reaches its conclusion. At this juncture, the looping structures have served their purpose, and the program proceeds to terminate its execution, having achieved its objectives through the power of iteration.
Looping structures in Python are indispensable tools for automating repetitive tasks, processing data, and performing iterative operations with efficiency and precision. By harnessing the capabilities of looping structures, Python programs can handle large datasets, implement complex algorithms, and tackle a wide range of computational challenges with ease and elegance.
For Loop: This is used to iterate over a sequence (like a list, tuple, or string).
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print("Current fruit :", fruit)
While Loop: This loop continues to execute as long as a certain condition is true.
count = 0
while count < 5:
print("Count is currently: ", count)
count += 1
In the for loop example, we’re iterating over each fruit in the list and printing it. In the while loop example, we’re printing the current count and then incrementing it by 1 until it’s no longer less than 5.
4. Control Flow Keywords
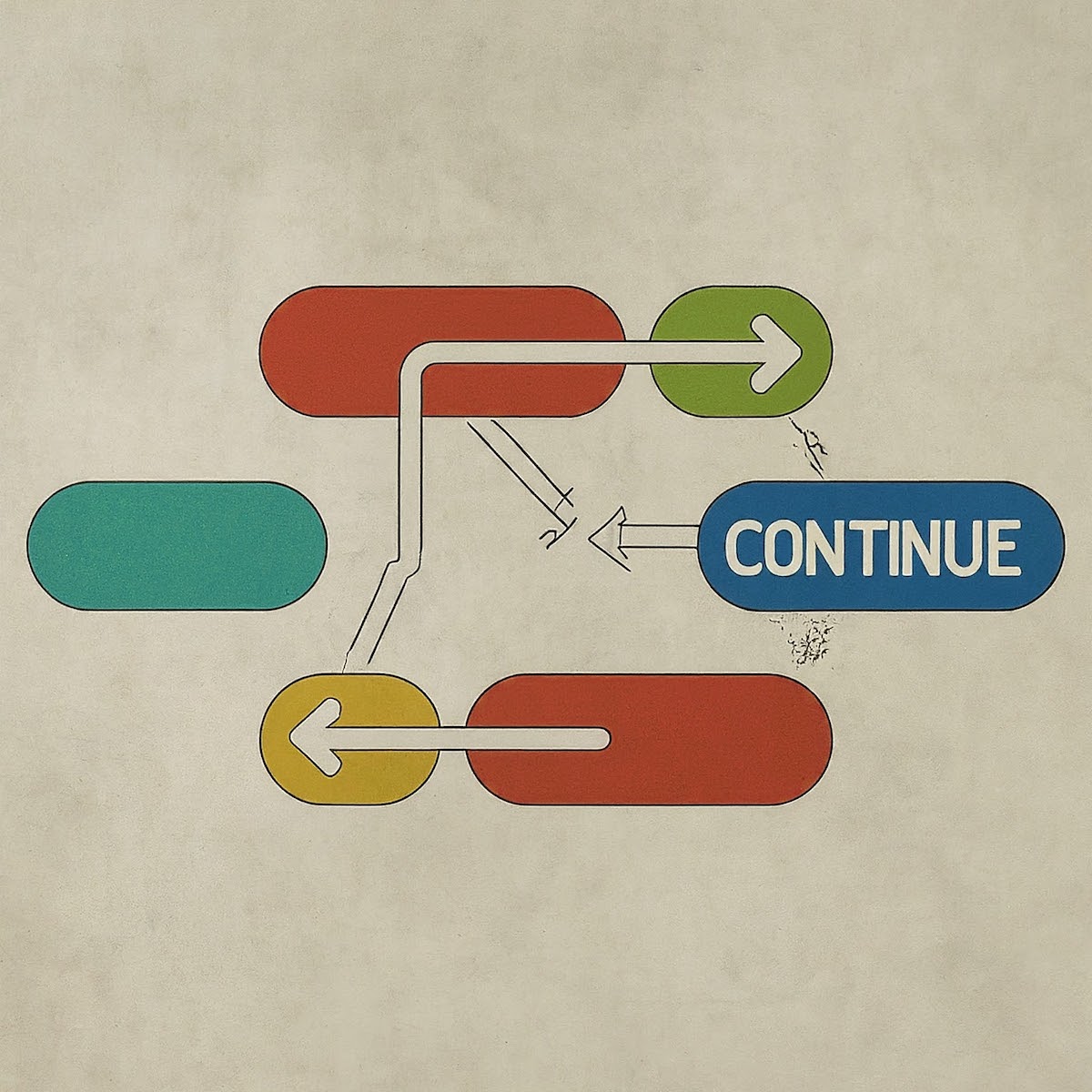
Python provides several keywords for altering the flow of control within a program. The break
keyword allows programmers to exit a loop prematurely, while the continue
keyword skips the remaining code in the current iteration of a loop and proceeds to the next iteration. Additionally, the pass
keyword serves as a placeholder for future code implementation, ensuring syntactic correctness without any action.
+-------------------+
| Control Flow |
| Keywords |
+-------------------+
|
v
Yes/No Decision
|
v
+---------+---------+
| | |
| Error | No Error|
| Occurred| |
| | |
+---------+---------+
| |
v v
+-------------------+
| Handle Error |
+-------------------+
|
v
+-------------------+
| Continue |
| Execution |
+-------------------+
|
v
+-------------------+
| End |
+-------------------+
Control flow keywords in Python provide mechanisms for altering the flow of execution within a program. Let’s explore some essential control flow keywords and their implications.
4.1 Yes/No Decision: Initiating Control Flow
At the outset, the program faces a crucial decision point – whether an error has occurred or not. This binary choice sets the stage for subsequent actions, dictating the flow of control based on the outcome.
4.2 Error Occurred: Handling Exceptions
In the event that an error is detected, the program enters the realm of exception handling. Here, specialized actions are taken to address the error and mitigate its impact on program execution. Control flow keywords such as try
and except
facilitate the graceful handling of exceptions, ensuring the stability and integrity of the program.
4.3 No Error: Continuing Execution
Conversely, if no error is detected, the program proceeds with uninterrupted execution. In this scenario, control flow continues seamlessly, and the program carries out its intended operations without the need for intervention.
4.4 Continue Execution: Resuming Program Flow
After handling any encountered errors, the program resumes its execution, proceeding with the remaining code. Control flow keywords like continue
allow the program to skip certain iterations or sections of code, enabling it to navigate through complex logic efficiently.
4.5 End: Culmination of Control Flow
As the program reaches its conclusion, it reflects on the journey of control flow. Whether errors were encountered or not, the program concludes with the assurance that it has navigated through potential obstacles with resilience and grace.
Control flow keywords in Python empower developers to write robust and reliable code that can gracefully handle various scenarios and exceptions. By leveraging these keywords effectively, Python programs can maintain stability, integrity, and efficiency, even in the face of adversity.
The following example demonstrates the use of these control flow keywords:
# Yes/No Decision: Initiating Control Flow
error_occurred = False
# Error Occurred: Handling Exceptions
try:
# Let's assume we have a potential error-causing operation here
if error_occurred:
raise ValueError("An error has occurred")
except ValueError as e:
print(f"Caught an error: {e}")
# Handle the error appropriately
else:
# No Error: Continuing Execution
print("No error occurred, continuing execution.")
# Continue Execution: Resuming Program Flow
for i in range(10):
# Using continue to skip certain iterations
if i % 2 == 0:
continue
print(i)
# Using break to exit a loop prematurely
for i in range(10):
if i > 5:
break
print(i)
# Using pass as a placeholder for future code implementation
def function_to_be_implemented():
pass
# End: Culmination of Control Flow
print("Program execution completed.")
The above includes examples of using try/except
for error handling, continue
and break
for loop control, and pass
as a placeholder for future code. The script concludes with a message indicating the end of program execution.
5. Nesting Control Flow Statements
Nesting control flow statements involves placing one control flow statement within another. This technique allows programmers to create complex decision-making structures or iterate over multidimensional data structures. Nested if-else
statements enable programmers to evaluate conditions within conditions, while nested loops facilitate the traversal of nested data structures or the implementation of multi-level iterations.
+----------------------------+
| Nesting Control Flow |
| Statements |
+----------------------------+
|
v
Yes/No Decision
|
v
+---------+---------+
| | |
| Condition TRUE | Condition FALSE
| | |
+---------+---------+
| |
v v
+---------+ +---------+
| Nested | | Nested |
| Control | | Control |
| Flow | | Flow |
|Statements| |Statements|
+---------+ +---------+
| |
v v
+-------------------+
| Conclude |
+-------------------+
|
v
+---------+
| End |
+---------+
Nesting control flow statements in Python allows for the creation of complex decision-making structures and iterative processes. Let’s delve into the process of nesting control flow statements and explore its implications.
5.1 Yes/No Decision: Setting the Stage
At the onset, the program faces a pivotal decision – whether a condition evaluates to true or false. This initial branching point determines the subsequent course of action, laying the groundwork for nested control flow.
5.2 Nested Control Flow: Expanding the Horizon
Upon evaluating the initial condition, the program may delve into nested control flow structures. Here, additional layers of decision-making or iteration unfold, as control flow statements are nested within one another to achieve intricate logic.
5.3 Conclusion: Reaching the Endpoint
As the program traverses through nested control flow structures, it eventually reaches a point of conclusion. Here, the final actions are executed, and the program prepares to terminate its execution.
5.4 End: Culmination of the Journey
As the journey through nested control flow statements draws to a close, the program reflects on the complexity and sophistication of its decision-making process. With each layer of nesting, the program navigates through increasingly intricate logic, demonstrating the versatility and power of control flow in Python.
Nesting control flow statements in Python enables developers to create sophisticated algorithms and tackle complex problem domains with finesse and clarity. By leveraging nested control flow, Python programs can exhibit robustness, flexibility, and scalability, empowering developers to craft elegant and efficient solutions to a wide range of challenges.
Here’s a simple example that uses nested if-else statements and nested loops:
# Nested If-Else Statements
def nesting_if_else(age):
if age >= 18:
if age >= 25:
print("You are an adult and can rent a car.")
else:
print("You are an adult but cannot rent a car.")
else:
print("You are a minor.")
# Nested Loops
def nesting_loops(matrix):
for row in matrix:
for element in row:
print(element, end=" ")
print()
# Test the functions
age = 20
nesting_if_else(age)
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
nesting_loops(matrix)
In the above example, nesting_if_else
function demonstrates nested if-else statements. It checks if a person is an adult (18 or older) and if they can rent a car (25 or older). The nesting_loops
function demonstrates nested loops by iterating over a 2D list (matrix) and printing its elements.
6. Error Handling with Control Flow
Error handling is an essential aspect of programming, and Python provides robust mechanisms for managing exceptions and errors. The try-except
block allows programmers to handle exceptions gracefully by catching and responding to errors within a specified block of code. The try-except-else-finally
block extends error handling capabilities by providing additional clauses for executing code under different circumstances, such as executing cleanup code regardless of whether an exception occurs.
+-------------------+
| Error Handling |
| with Control Flow |
+-------------------+
|
v
Yes/No Decision
|
v
+---------+---------+
| | |
| Error | No Error|
| Occurred| |
| | |
+---------+---------+
| |
v v
+-------------------+
| Handle Error |
+-------------------+
|
v
+---------+
| End |
+---------+
6.1 Yes/No Decision: Assessing Error Occurrence
At the outset, the program encounters a pivotal decision point – whether an error has occurred or not. This binary choice sets the stage for subsequent actions, dictating the flow of control based on the outcome.
6.2 Error Occurred: Navigating the Path of Exception
In the event that an error is detected, the program diverges into the realm of exception handling. Here, specialized actions are taken to address the error and mitigate its impact on program execution. Exception handling mechanisms, such as try-except
blocks, come into play to intercept and handle the error gracefully.
6.3 No Error: Proceeding Uninterrupted
Conversely, if no error is detected, the program proceeds along the path of uninterrupted execution. In this scenario, control flow continues seamlessly, and the program carries out its intended operations without the need for intervention.
6.4 Handle Error: Resolving and Recovering
When an error is encountered, the program enters the realm of error handling, where it employs various strategies to resolve and recover from the error. This may involve logging the error for future analysis, providing informative error messages to the user, or executing alternative code paths to ensure program continuity.
6.5 End: Conclusion of Error Handling
As the program reaches its conclusion, it reflects on the journey of error handling with control flow. Whether errors were encountered or not, the program concludes with the assurance that it has navigated through potential obstacles with resilience and grace.
Error handling with control flow in Python empowers developers to write robust and reliable code that can gracefully handle unforeseen circumstances. By integrating error handling mechanisms seamlessly into the flow of control, Python programs can maintain stability and integrity, even in the face of adversity.
def divide_numbers(a, b):
try:
# Attempt to execute the code within the try block
result = a / b
except ZeroDivisionError as e:
# Handle the ZeroDivisionError exception
print(f"Error: {e}. Cannot divide by zero.")
except TypeError as e:
# Handle the TypeError exception
print(f"Error: {e}. Please enter valid numbers.")
else:
# Execute this block if no exceptions occurred in the try block
print(f"Result: {result}")
finally:
# Execute this block regardless of whether an exception occurred or not
print("Program execution completed.")
# Request user input
num1 = input("Enter the first number: ")
num2 = input("Enter the second number: ")
# Call the function with user input
divide_numbers(float(num1), float(num2))
The above example demonstrates error handling with control flow in Python by taking two numbers as input and attempting to divide the first number by the second number. If a ZeroDivisionError or TypeError occurs, the script will handle the exception accordingly and print an appropriate error message. If no exceptions occur, the script will print the result of the division. Finally, the script will print a message indicating that the program execution has completed.
7. Advanced Control Flow Techniques
Python offers advanced control flow techniques that enhance code readability and conciseness. List comprehensions provide a concise syntax for generating lists based on existing sequences or iterables, offering an elegant alternative to traditional looping structures. Generator expressions, on the other hand, enable the creation of generators, which produce values lazily, conserving memory and improving performance in certain scenarios. Understanding and leveraging these advanced control flow techniques can significantly enhance productivity and code efficiency in Python programming.
+-----------------------+
| Advanced Control Flow |
| Techniques |
+-----------------------+
|
v
Yes/No Decision
|
v
+---------+---------+
| | |
| List | Generator|
|Compreh-| Express- |
|ensions | ions |
+---------+---------+
| |
v v
+-------------------+
| Utilize |
| Techniques |
+-------------------+
|
v
+-------------------+
| End |
+-------------------+
Advanced control flow techniques in Python offer sophisticated approaches to enhance code readability, efficiency, and conciseness. Let’s explore two such techniques: List Comprehensions and Generator Expressions.
7.1 Yes/No Decision: Embracing Advanced Techniques
At the onset, the program faces a pivotal decision – whether to employ advanced control flow techniques. This decision allows us to use list comprehensions and generator expressions to make our code run smoother.
7.2 List Comprehensions: Streamlining List Generation
List comprehensions provide a concise and expressive syntax for generating lists based on existing sequences or iterables. List comprehensions make code easier to read and shorter by turning a regular loop into just one line.
7.3 Generator Expressions: Enhancing Efficiency
Generator expressions offer a memory-efficient and performance-optimized approach for generating values lazily. Unlike list comprehensions, which store everything in memory at once, generator expressions produce values one by one, saving memory and making things faster.
7.4 Utilize Techniques: Maximizing Productivity
While the program goes through these advanced techniques, it uses list comprehensions and generator expressions to make the code run better. By leveraging these techniques effectively, Python programs can achieve greater productivity, readability, and efficiency, leading to improved code quality and maintainability.
7.5 End: Culmination of Advanced Techniques
Upon completion of the utilization of advanced control flow techniques, the program reaches its conclusion. Now, the program has used list comprehensions and generator expressions to make the code better, neater, and more efficient.
Advanced control flow techniques in Python empower developers to write elegant, efficient, and maintainable code that can tackle complex tasks with ease. By mastering techniques such as list comprehensions and generator expressions, Python programmers can elevate their coding skills and unlock new possibilities for innovation and optimization.
Ok let’s implement a simple Python script that demonstrates the use of list comprehensions and generator expressions:
# List Comprehensions: Streamlining List Generation
# Here we will create a list of squares of numbers from 1 to 10 using list comprehension
squares_list = [i**2 for i in range(1, 11)]
print("List Comprehension: ", squares_list)
# Generator Expressions: Enhancing Efficiency
# Here we will create a generator that yields the squares of numbers from 1 to 10
squares_generator = (i**2 for i in range(1, 11))
print("Generator Expression: ")
for value in squares_generator:
print(value)
# Utilize Techniques: Maximizing Productivity
# Here we will use list comprehension to filter out the even squares and generator expression to get the sum of the odd squares
even_squares = [value for value in squares_list if value % 2 == 0]
print("Even squares using List Comprehension: ", even_squares)
odd_squares_sum = sum(value for value in squares_generator if value % 2 != 0)
print("Sum of odd squares using Generator Expression: ", odd_squares_sum)
In the above example, we first use a list comprehension to generate a list of squares. Then, we use a generator expression to lazily generate the same sequence of squares. We then utilize these techniques to filter out even squares using a list comprehension and calculate the sum of odd squares using a generator expression. This demonstrates how these advanced control flow techniques can be used to enhance code readability, efficiency, and conciseness.
8. Conclusion
Mastering by practicing the control flow techniques in Python is essential for writing efficient, reliable, and maintainable code. From conditional statements to advanced control flow techniques like list comprehensions and generator expressions, Python offers a rich set of tools to manage program flow effectively.
In essence, control flow techniques in Python form the backbone of programming. It empowers the developers to write code that is expressive, efficient, and reliable. By mastering these techniques, programmers can build powerful and versatile applications that meet the demands of today’s dynamic software landscape. Happy coding!
I like this blog it’s a master piece! Glad I discovered this ohttps://69v.topn google.Raise blog range